When Apple released the iPhone SDK in the spring of 2008, many developers were ecstatic about the development potential of the iPhone. However, they were also frustrated with the fact that they had to develop applications in Objective-C which required a lot of low-level memory management and header file declarations. This didn’t seem to stop developers, though—over 100,000 applications have been developed since then. Still, the frustration was very real, especially among developers used to the development experience provided by Microsoft with C# and .NET. Now the mobile community has been rocked by the release of MonoTouch, which allows native iPhone development to be performed in C# and .NET.
What is MonoTouch?
MonoTouch provides the ability to develop and compile native iPhone applications in .NET. While this may seem surprising to many, Mono is not new to the iPhone. It’s been around for over a year now, working behind the scenes in the popular commercial game engine, Unity3d. There are hundreds of Unity3d-powered apps in the app store, and now the announcements of apps based on MonoTouch being accepted into the App Store are beginning to trickle in. While many have said that it would be impossible to bring a platform like .NET to the iPhone because of Apple’s restriction to dynamic code generation, Novell worked around this by eliminating the JIT (Just In Time) compiler and replacing it with a static pre-compiler.
MonoTouch is a commercial product from Novell, which is also the sponsor of the entire Mono project. While Mono is free, open-source software, MonoTouch costs between $399 and $999 per developer, depending on the version purchased. Pure Microsoft developers may find it difficult to transition to MonoTouch development. As with the Apple’s iPhone SDK and development tools, MonoTouch is only available for the Mac.
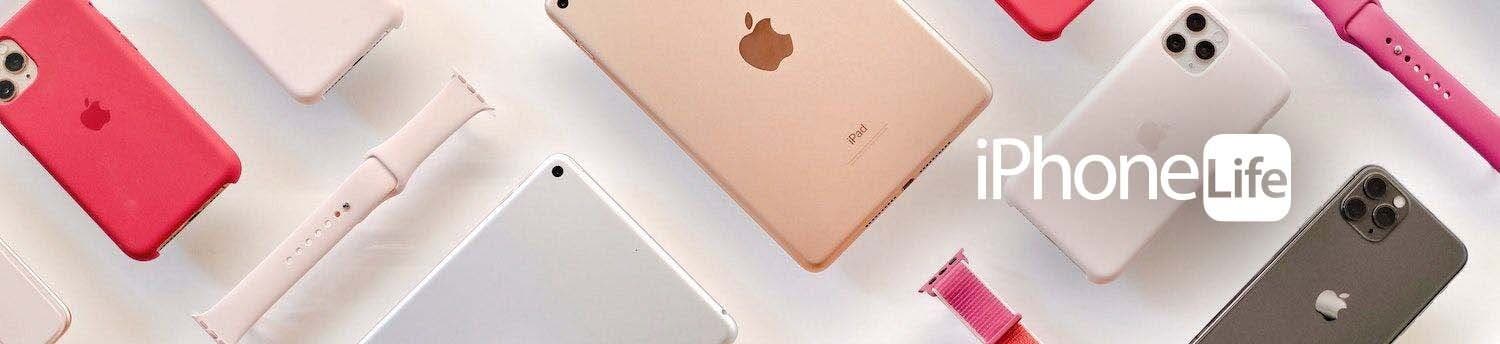
Advantages of MonoTouch
For most .NET developers, the single most important advantage of MonoTouch is the ability to leverage their existing experience and even existing code when developing for the iPhone. Even for developers who aren’t as familiar with .NET, MonoTouch can still provide some significant advantages.
C# 3.0
C# is a simple, rich, modern, general-purpose, object-oriented programming language with many features to improve programmer productivity. In addition to those provided by traditional object oriented languages, C# offers many powerful new constructs, including type safe generic types, expression trees, lambda methods, extension methods, built-in properties, and event systems. The syntax of the language allows for clean and simple configuration of objects, as shown here:
var slider = new UISlider (new RectangleF (174f, 12f, 120f, 7f)){
BackgroundColor = UIColor.Clear,
MinValue = 0f,
MaxValue = 100f,
Continuous = true,
Value = 50f,
Tag = kViewTag
};
slider.ValueChanged += delegate {
Console.WriteLine (“New value {0}”, slider.Value);
};
LINQ
Language Integrated Query (LINQ) provides native data querying capabilities that allow developers to query data using both strongly-typed queries and strongly-typed results with syntax that is common across disparate data types. (MonoTouch supports both LINQ to Objects and LINQ to XML.) LINQ can improve developer productivity through the benefits of IntelliSense and compile-time error checking.
Automatic Memory Management
MonoTouch provides a garbage collector that automatically handles all of the low-level memory management tasks associated with releasing objects. This improves developer productivity by both avoiding memory leaks and simultaneously reducing the amount of code that needs to be written in a given application.
iPhone APIs
MonoTouch provides bindings to the entire collection of CocoaTouch Objective-C iPhone 3.1 APIs (with the exception of CoreData). This means all of the native iPhone classes in CoreAnimation/Quartz, CoreLocation, GameKit, StoreKit, UIKit and more are accessible as .NET objects. Objective-C delegates are exposed to C# applications in several ways, including either traditional Obj-C style or new C# style.
.NET APIs
MonoTouch includes a subset of the .NET Framework that consists of APIs that make sense in the context of the iPhone’s hardware and software capabilities. This includes most of what is supported in the Silverlight foundation. In addition, it includes System.IO, System.XML, System.XML.Linq, System.JSON, System.Web.Services, System.Threading, RegularExpressions, and a subset of System.Data.
Existing .NET Libraries
Because MonoTouch supports such a significant subset of the full .NET framework, many third-party .NET libraries are consumable by MonoTouch with minimal refactoring. In addition, existing .NET code or business logic can often be reused on the iPhone. Some libraries that other MonoTouch developers are already using can be found on the MonoTouch Wiki (wiki.monotouch.net).
Third Party Obj-C Libraries
Since there is a growing collection of native iPhone libraries in Objective-C, MonoTouch not only provides a mechanism to P/Invoke unmanaged native libraries, but also a tool that can automatically generate native bindings through the creation of a simple API Definition file. A collection of these bindings can be found at: code.google.com/p/btouch-library.
MonoDevelop Integration
MonoTouch requires an Intel-based Mac OS X and Apple’s iPhone SDK, so development must be done on a Mac; it does not support Windows-based development. While many developers will lament the absence of Visual Studio, MonoDevelop provides a very compelling IDE with rich IntelliSense and even integrated source code management.
Interface Builder Integration
Interface Builder is Apple’s User Interface Design tool, and MonoTouch takes integration one step further than even what Apple has provided with XCode. Rather than requiring developers to manually bind any outlets, MonoTouch automatically creates bindings on the interface in a C# code-behind partial class.
Integrated Debugger
MonoTouch offers a soft-debugger integrated into the MonoDevelop IDE and supports step-through debugging on either the simulator or a physical device. One of the greatest features of the debugger is that it is network based which allows device debugging over Wi-Fi (or even over the Internet and around the world if the development machines are configured to be externally accessible).
Limitations of MonoTouch
The MonoTouch team released the debugger just over a month after the initial product release. The debugger might have eliminated the biggest weakness of MonoTouch, but that doesn’t mean though that there aren’t limitations to MonoTouch.
No Dynamic Code Generation
Because Apple does not allow applications to generate code dynamically on the iPhone, MonoTouch does not support any form of dynamic code generation. Therefore, there is no support for System.Reflection.Emit, System.Runtime.Remoting, dynamic type creation, COM bindings, the JIT engine, or Metadata verifier.
Limited Generics Support
Since all code on the iPhone is statically compiled during the build instead of being compiled on demand by the JIT engine (as is the case in standard .NET or traditional Mono), MonoTouch has certain limitations with Generics. Specifically, neither Generic Virtual Methods nor P/Invokes in Generic Types are supported.
What does this mean to both the iPhone and .NET developer communities?
Only time will tell whether MonoTouch will have a significant impact on iPhone development. I believe that it will be a catalyst that helps continue the acceleration of iPhone development. In addition, I think it will help bring the very significant amount of C#/.NET developer experience to the iPhone, along with existing libraries and codebases. Enterprises that have existing mobile line-of-business applications developed in the .NET Compact Framework for Windows Mobile devices will see that this is a way to reuse existing investments as they begin to explore supporting the iPhone. Overall, the fusion of both the Apple and Microsoft worlds has the potential to be great for both companies and both communities, as well as for Novell and the Mono Project.
Other .NET Development Alternatives: ComponentOne Studio for iPhone
MonoTouch allows the development of native iPhone applications in .NET, but requires a Mac and does not integrate with Visual Studio. ComponentOne has released a suite of ASP.NET controls that do integrate with Visual Studio and deliver the iPhone User Experience as either a Web or Hybrid application. While there are a number of similar open source frameworks for developing Web apps that look and feel like native iPhone applications, none of them are as cleanly put together and include ASP.NET, Visual Studio integration, simple installation, documentation, or sample code.
ComponentOne’s Calendar Control: Even though it is based in a WebView inside Safari, it nearly perfectly replicates the native calendar experience.
Many of the ComponentOne controls are quite amazing and gorgeous for HTML and CSS-based controls. For instance, the Calendar control is virtually indistinguishable from the native calendar functionality. The CoverFlow also works very well, although performance is slightly less smooth on any device less than an iPhone 3GS. Experienced ASP.NET developers will love how easy it is to integrate these controls with back-end data sources using data binding. They will also appreciate how much it speeds up the development of apps that access back-end data. However, the suite is rough around the edges in some places and does not perform as well as some free libraries. For example, the fixed header scrolling and navigation bar slide-in animation are decidedly jerky even on a powerful 3GS device.
Even though this suite is pricy at $800.00, it’s the perfect solution for many development efforts. And since its requirements and development paradigm are significantly different from MonoTouch, I don’t believe the two products will compete with each other. Consider them complimentary products that are used for different purposes to develop different types of iPhone applications.