How to Record Video on iPhone the Fastest Way
What is QuickTake video? It's the fastest and easiest way of recording video on your iPhone!


How do you take a video in picture mode on an iPhone? Use Apple's QuickTake, the fastest way to record a video on the iPhone.
Related: How to Screen Record with Audio on an iPhone & iPad
Why You’ll Love This Tip
- Record video on your iPhone without leaving photo mode.
- Makes recording video quick and easy, so you don't miss important moments.
How to Record Video on iPhone with QuickTake
When you find the perfect moment for a video, you don’t always have time to switch between Photo and Video mode on your iPhone. This tip for the iPhone 11, 11 Pro, 11 Pro Max, and later will show you how to take a video on an iPhone right from Photo mode. To learn more about the Camera app and other iPhone apps, check out our Tip of the Day newsletter.
- Open the Camera app.
- In Photo mode, press and hold the Shutter button; your iPhone will begin video recording.
- You can drag the Shutter button to the right to temporarily lock your camera into Video mode.
- When you're finished, tap the red square or release the Shutter button (if you didn't slide it to the right) to stop recording.
When you’re finished recording, your Camera app will return to Photo mode.
That’s it! Unfortunately, this trick only works on newer iPhones. But if you can't use it, then this method of taking photos while video shooting is great for capturing surprise shots at soccer matches or catching a pet in a cute moment without having to fumble about with Camera settings. The Camera app is truly versatile, and perfect for capturing life’s most precious and exciting moments. Next, learn how to reverse videos on iPhone.
Every day, we send useful tips with screenshots and step-by-step instructions to over 600,000 subscribers for free. You'll be surprised what your Apple devices can really do.
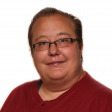
Tamlin Day
Tamlin Day is a feature web writer for iPhone Life and a regular contributor to iPhone Life magazine. A prolific writer of tips, reviews, and in-depth guides, Tamlin has written hundreds of articles for iPhone Life. From iPhone settings to recommendations for the best iPhone-compatible gear to the latest Apple news, Tamlin's expertise covers a broad spectrum.
Before joining iPhone Life, Tamlin received his BFA in Media & Communications as well as a BA in Graphic Design from Maharishi International University (MIU), where he edited MIU's literary journal, Meta-fore. With a passion for teaching, Tamlin has instructed young adults, college students, and adult learners on topics ranging from spoken word poetry to taking the perfect group selfie. Tamlin's first computer, a Radioshack Color Computer III, was given to him by his father. At 13, Tamlin built his first PC from spare parts. He is proud to put his passion for teaching and tech into practice as a writer and educator at iPhone Life.