How to Unzip Files on the iPhone in the Files App


f you want to know how to unzip files on the iPhone or iPad, you can extract that zip file straight from the Files app! This is great news, since in the past unzipping a file on the iPhone required third party software. Now, you can unzip files and view them inside the Files app! Let’s dive in with how to extract a zip file on the iPhone.
Related: How to Browse Your iCloud Drive Documents in the Files App
How to Open Zip Files on the iPhone
- Open the Files app.
- Navigate to the folder where you’ve saved the file you want to unzip. By default, this should be your Downloads folder.
- Tap the zipped file and wait a moment for the file to unzip.
- Tap the unzipped file to view the contents.
That’s it! What used to be a complicated is now so simple. Being able to create PDFs on the iPhone, send them, and open them as zip files, along with pictures and other media, makes the iPhone an even more robust productivity tool. Just be sure to check the size of a file before you unzip it, and to only unzip files from trusted websites or contacts.
Top image credit: SFIO CRACHO / Shutterstock.com
Every day, we send useful tips with screenshots and step-by-step instructions to over 600,000 subscribers for free. You'll be surprised what your Apple devices can really do.
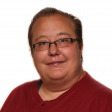
Tamlin Day
Tamlin Day is a feature web writer for iPhone Life and a regular contributor to iPhone Life magazine. A prolific writer of tips, reviews, and in-depth guides, Tamlin has written hundreds of articles for iPhone Life. From iPhone settings to recommendations for the best iPhone-compatible gear to the latest Apple news, Tamlin's expertise covers a broad spectrum.
Before joining iPhone Life, Tamlin received his BFA in Media & Communications as well as a BA in Graphic Design from Maharishi International University (MIU), where he edited MIU's literary journal, Meta-fore. With a passion for teaching, Tamlin has instructed young adults, college students, and adult learners on topics ranging from spoken word poetry to taking the perfect group selfie. Tamlin's first computer, a Radioshack Color Computer III, was given to him by his father. At 13, Tamlin built his first PC from spare parts. He is proud to put his passion for teaching and tech into practice as a writer and educator at iPhone Life.